You can use the Import-CSV or Get-Content cmdlets with the foreach loop in PowerShell to read the CSV file line by line.
The following methods show how you can do it with syntax.
Method 1: Using Import-CSV to read CSV line-by-line
Import-CSV -Path $csvFilePath |foreach-object {
$line = $_
Write-Output $line
}
This example will read the CSV file line by line and output the CSV content on the console.
Method 2: Using the Get-Content to read CSV file line by line
Get-Content -Path $csvFilePath |foreach-object {
$line = $_
Write-Output $line
}
This example will read the CSV file line by line using the Get-Content cmdlet.
The following examples show how you can use these methods.
How to Read CSV File Line by Line Using Import-CSV in PowerShell
The following PowerShell script shows how you can read a csv file using the Import-CSV cmdlet.
# specify the csv file path $csvFilePath = "C:\temp\log\processData.csv" # Import csv file and iterate line by line Import-CSV -Path $csvFilePath |foreach-object { $line = $_ Write-Output $line }
Output:
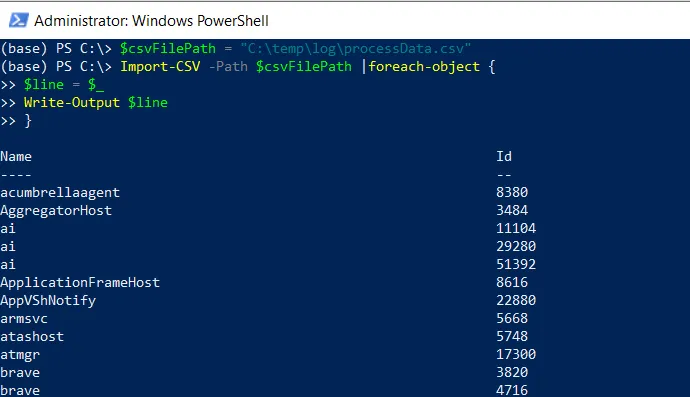
In this script, we define a $csvFilePath variable to store the path of the CSV file.
We then use the Import-CSV cmdlet to import the specified $csvFilePath CSV file and pipe the array of objects to the ForEach-Object cmdlet.
The Foreach-Object command iterates over each line of the CSV file. Inside the loop, you can process each line as needed.
In this example, we use the Write-Output cmdlet to output the line.
After running this script, the PowerShell imports the CSV file iterates over line by line, and displays it on the console.
How to Read CSV File Line by Line Using Get-Content in PowerShell
The following PowerShell script shows how you can read a csv file using the Get-Content cmdlet.
# specify the csv file path $csvFilePath = "C:\temp\log\processData.csv" # read csv file and iterate line by line Get-Conte -Path $csvFilePath |foreach-object { $line = $_ Write-Output $line }
Output:
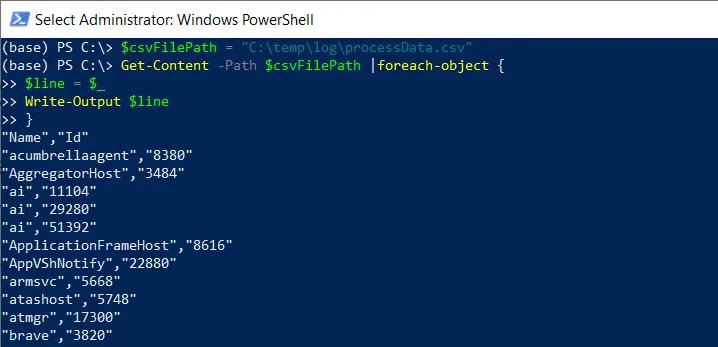
In this script, we define a $csvFilePath variable to store the path of the CSV file.
We then use the Get-Content cmdlet to import the specified $csvFilePath CSV file and pipe the array of objects to the ForEach-Object cmdlet.
The Foreach-Object command iterates over each line of the CSV file. Inside the loop, you can process each line as needed.
In this example, we use the Write-Output cmdlet to output the line.
After running this script, the PowerShell imports the CSV file iterates over line by line, and displays it on the console.
Conclusion
I hope the above article on reading the CSV file line by line using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.