In PowerShell, the Where-Object cmdlet can be used to filter elements in the list or array based on specific conditions.
The following methods show how you can do it with syntax.
Method 1: Filtering elements from a list using Where-Object
$languages = @("Python", "C#", "C", "C++", "VB", "Java", "Go")
$result = $languages | Where-Object { $_ -ne "Java"}
$result
In this example, it will exclude the “Java” language from the list and return the elements in the $languages.
Method 2: Filter elements from a list
$list = @(10, 20, 30, 40, 50, 60, 70, 80, 90, 100)
$result = $list | Where-Object { $_ -gt 50 }
$result
In this example, it will filter elements from a list and return the element greater than 50.
Method 3: Filter elements from a list of custom objects
$employees = @(
[PSCustomObject]@{ Name = "Alex"; Age = 30; Department = "IT" },
[PSCustomObject]@{ Name = "Gary"; Age = 35; Department = "Sales" },
[PSCustomObject]@{ Name = "Ricky"; Age = 38; Department = "IT" }
)
$itEmployees = $employees | Where-Object { $_.Department -eq "IT" }
$itEmployees
In this example, it will return the list of employees in the IT department.
The following examples show how you can use these methods.
Filtering Elements from a List Using Where-Object
The following PowerShell script shows how you can do it.
# define a list of languages $languages = @("Python", "C#", "C", "C++", "VB", "Java", "Go") # Filter the elements in the list to exclude "Java" and get other languages $result = $languages | Where-Object { $_ -ne "Java"} # Output the list $result
Output:
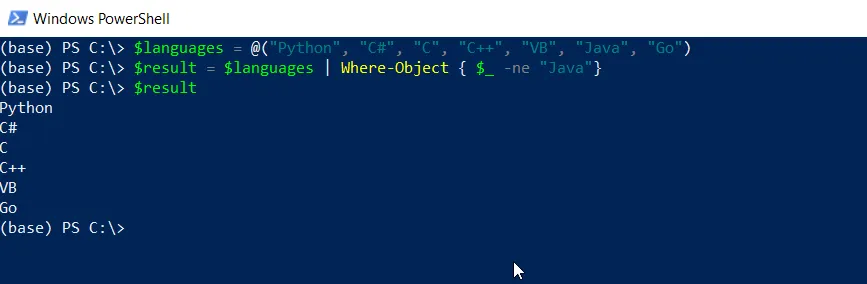
In this example, we define a $languages
variable that contains a list of programming languages.
The $languages
pipe to the Where-Object cmdlet to filter elements based on the condition specified { $_ -ne "Java"}
. It returns the list of elements excluding “Java” and stores them in the $result
variable.
Finally, we print the list of elements.
Using Where-Object in List to Filter Elements
Let’s consider an example, we have a list of numbers. The objective is to get the list of numbers greater than 50.
The following PowerShell script shows how you can do it.
# Create a list of numbers $list = @(10, 20, 30, 40, 50, 60, 70, 80, 90, 100) # Filter the element from a list greater than 50 $result = $list | Where-Object { $_ -gt 50 } # Outputs the result $result
Output:
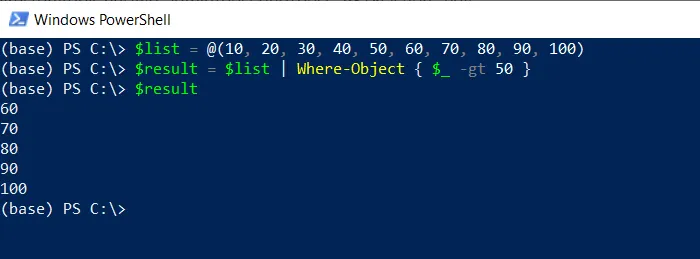
In this PowerShell example, we define a $list that contains the list of numbers.
We then pipe the $list to the Where-Object cmdlet to filter elements greater than 50 and store the result in the $result variable.
Finally, it outputs the result.
Using PowerShell Where-Object in List of Custom Objects
You can use the Where-Object cmdlet in PowerShell to filter elements from a list of custom objects. Here’s how you can do it.
# Create a list of custom objects $employees = @( [PSCustomObject]@{ Name = "Alex"; Age = 30; Department = "IT" }, [PSCustomObject]@{ Name = "Gary"; Age = 35; Department = "Sales" }, [PSCustomObject]@{ Name = "Ricky"; Age = 38; Department = "IT" } ) # Filter the list to get employees in the IT department $itEmployees = $employees | Where-Object { $_.Department -eq "IT" } $itEmployees
Output:
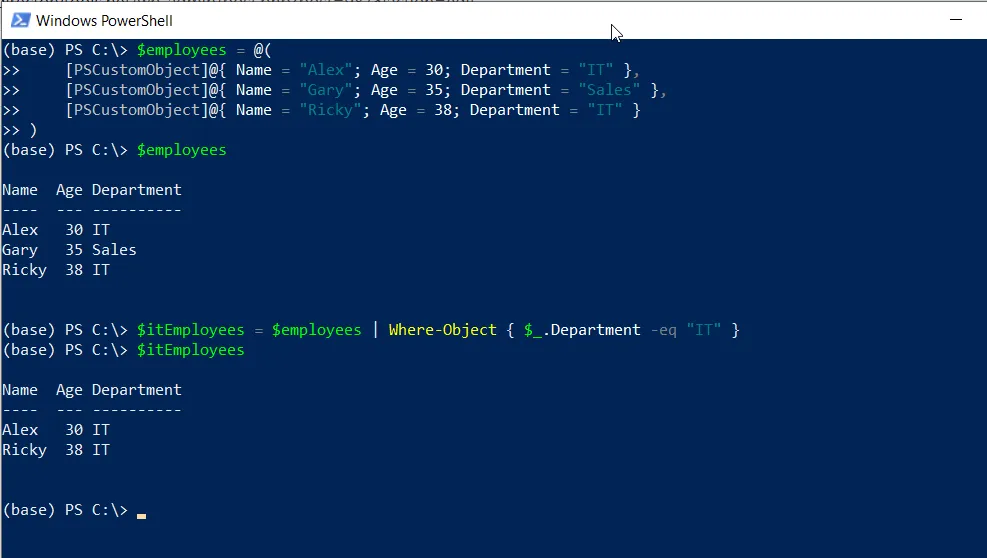
In this example, we have created a list of custom objects using [PSCustomObject]
and stored them in the $employees
variable.
We use the Where-Object
to filter the employees based on their Department property and select only those in the “IT” department.
The resulting $itEmployees
variable contains the filtered list containing employees from the “IT” department.
Conclusion
I hope the above article on using the PowerShell Where-Object cmdlet in List is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.