To replace a string in a file, you can use the Get-Content
cmdlet to read the file content, followed by the -replace
operator to perform replacement. It replaces every occurrence of a string in a file. You can also the replace() method to replace a string in a file.
Method 1: Using -replace operator with Get-Content cmdlet to replace every occurrence of a string in a file
(Get-Content -Path C:\temp\file1.txt) -replace "old_string", "new_string"
Method 2: Using Replace() method with the Get-Content cmdlet to replace every occurrence of a string in a file
(Get-Content -Path C:\temp\file1.txt).Replace( "old_string", "new_string")
Both of these methods replace every occurrence of the string old_string with a “new_string” in the file named file1.txt.
The following example demonstrates the practical application to replace a string in a file in PowerShell.
Using -replace Operator to Replace Every Occurrence of String in a File in PowerShell
Let’s consider an example, we have a file named “file1.txt
” that contains the below information.
This is test file.
Let’s use the Get-Content
cmdlet to read the file contents and store the information in the $file_content
variable. The $old_string
variable contains the string you want to replace and the $new_string
variable contains the string to replace with.
After reading the text file, it uses the -replace
operator to provide $old_string
and $new_string
variable to replace every occurrence of a string in a file and save the modified file data in the $new_content
variable.
The Set-Content
cmdlet in PowerShell is used to save the modified information to the file.
# Define the file path $file_path = "C:\temp\file1.txt" # Read the contents of the file $file_content = Get-Content -Path $file_path -Raw # Define the string you want to replace $old_string = "test" # Define the new string to replace with $new_string = "demo" # Replace every occurrence of the old string with the new string $new_content = $file_content -replace $old_string, $new_string # Write the modified content back to the file Set-Content -Path $file_path -Value $new_content
The output of the above PowerShell script replaces every occurrence of the string “test” with the “demo” string in a file.
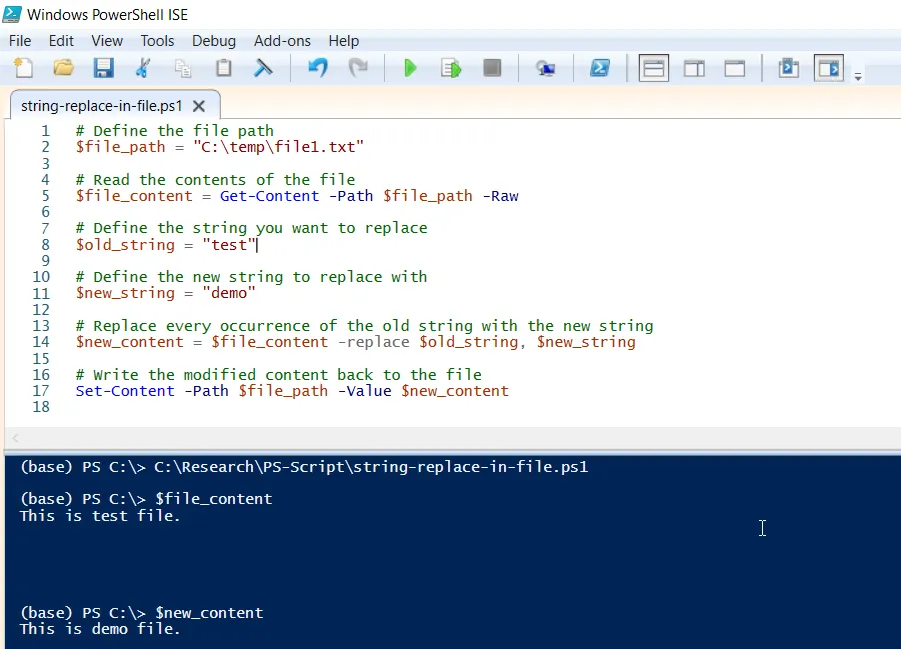
This script reads the contents of the file, replaces every occurrence of the old string with the new string, and then writes the modified content back to the file.
Using the Replace() Method to Replace Every Occurrence of String in a File in PowerShell
Let’s consider an example, we have a file named “file1.txt
” that contains the below information.
This is test file.
Let’s use the Get-Content
cmdlet to read the file contents and store the information in the $file_content
variable. The $old_string
variable contains the string you want to replace and the $new_string
variable contains the string to replace with.
After reading the text file, it uses the Replace()
method that takes $old_string
and $new_string
variable as inputs. It replaces every occurrence of a string in a file, and saves the modified file data in the $new_content
variable.
The Set-Content
cmdlet in PowerShell is used to save the modified information to the file.
# Define the file path $file_path = "C:\temp\file1.txt" # Read the contents of the file $file_content = Get-Content -Path $file_path -Raw # Define the string you want to replace $old_string = "test" # Define the new string to replace with $new_string = "demo" # Replace every occurrence of the old string with the new string $new_content = $file_content.Replace($old_string, $new_string) # Write the modified content back to the file Set-Content -Path $file_path -Value $new_content
The output of the above PowerShell script replaces the old_string in a file with new_string
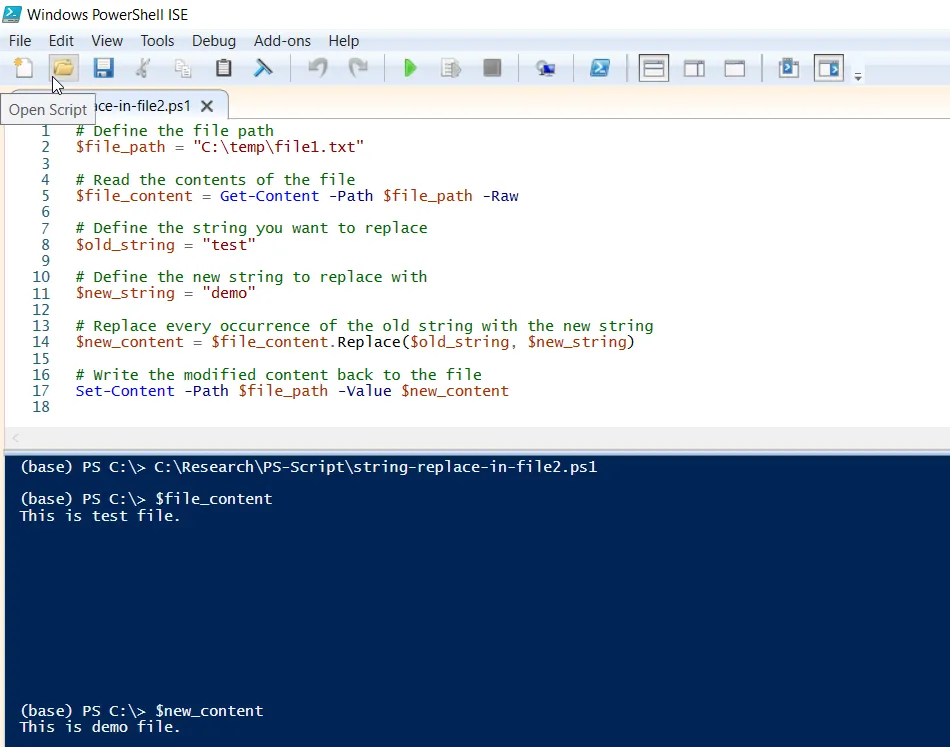
We can see the $new_content
variable has the new content after replacing the “test” with the “demo” string in a file in PowerShell.
Conclusion
I hope the above article on how to replace the string in a file using PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.