In PowerShell, you can use the Select-Object cmdlet along with calculated properties to select the nested properties. The calculated properties are useful when you want to retrieve specific information from nested objects.
The following methods show how you can do it with syntax.
Method 1: Selecting nested properties
$processList = Get-Process | Select-Object -Property Name, @{Name="MainModuleFileNameLength"; Expression={$_.MainModule.FileName.Length}}
$processList
This example will return the process name and MainModuleFileName
as nested properties from the object.
Method 2: Selecting nested properties from the custom object
$object = @(
[PSCustomObject]@{Name = "Gary"; Address = @{City = "New York"; Zip = "10001"}}
)
# Select nested properties
$selectedProperties = $objects | Select-Object -Property Name, @{Name="City"; Expression={$_.Address.City}}, @{Name="Zip"; Expression={$_.Address.Zip}}
$selectedProperties
This example will return the nested properties of the custom object.
The following examples show how you can use these methods.
Get Nested Properties Using Select-Object in PowerShell
You can use the Select-Object cmdlet in PowerShell to select the nested properties.
# Get processes and select nested properties $processList = Get-Process | Select-Object -Property Name, @{Name="MainModuleFileNameLength"; Expression={$_.MainModule.FileName.Length}} # Output the processes with the selected nested properties Write-Output $processList
Output:
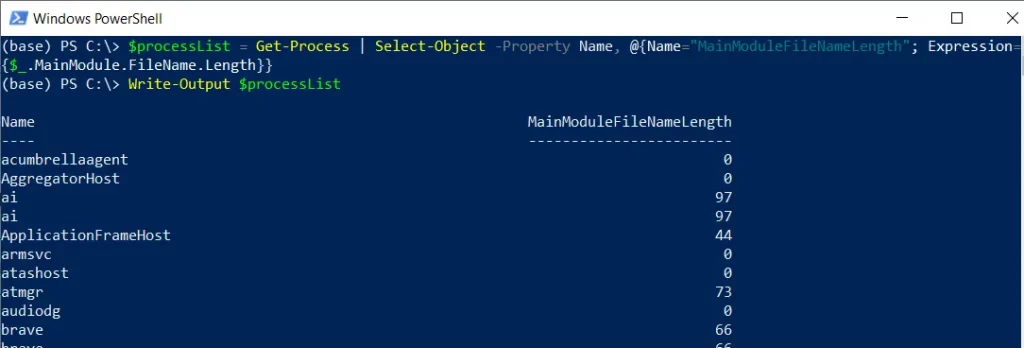
In this example, the Get-Process cmdlet gets the processes and pipes them to the Select-Object cmdlet to select the property and nested property. In this example, the length of the main module’s file name is a nested property.
It stores the processes with the selected nested properties in the $processList
variable.
Finally, we use the Write-Output cmdlet to output the processes with the selected nested properties.
Selecting Nested Properties from the Custom Object
You can also use the Select-Object cmdlet to extract nested properties from the custom object. Here’s how you can do it.
# create a custom object with nested properties (City, Zip) $object = @( [PSCustomObject]@{Name = "Gary"; Address = @{City = "New York"; Zip = "10001"}} ) # Select nested properties $selectedProperties = $object | Select-Object -Property Name, @{Name="City"; Expression={$_.Address.City}}, @{Name="Zip"; Expression={$_.Address.Zip}} # Output the custom object with the selected nested properties Write-Output $selectedProperties
Output:
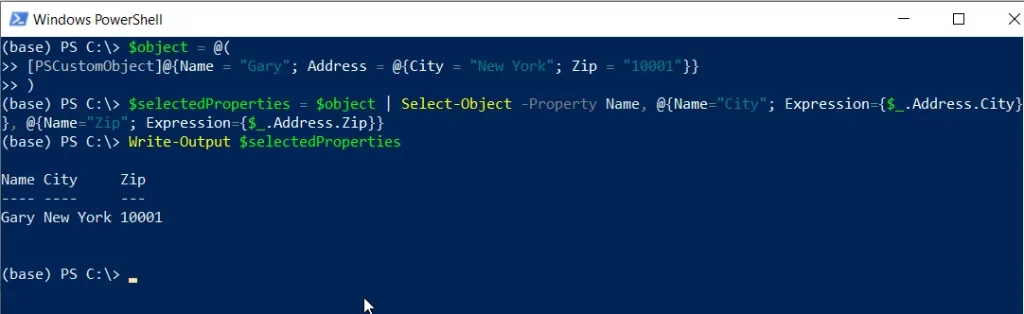
In this example, we create a custom object $object
using the [PSCustomObject]
that contains the nested properties, such as City and Zip.
We then pipe the $object
to the Select-Object cmdlet to select the property and nested properties ( City and Zip) and store the result in the $selectedProperties
variable.
Finally, we use the Write-Output cmdlet to output the custom object with the nested properties.
Conclusion
I hope the above article on getting the nested properties using the Select-Object cmdlet in PowerShell is helpful to you.
You can find more topics about Active Directory tools and PowerShell basics on the ActiveDirectoryTools home page.